2. productCartList: This component is having all the products which user select to add in cart.
this component is sending product information through message channel
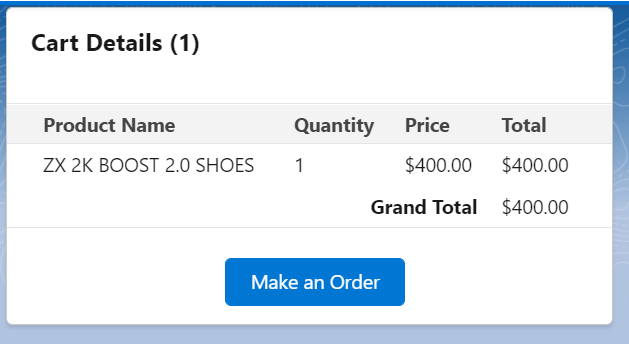
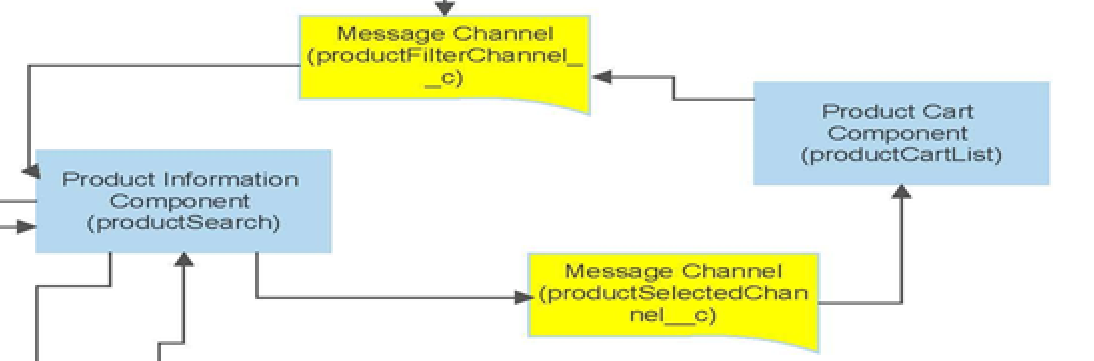
productCartList.JS
import { LightningElement, api,wire, track } from "lwc";
import ACCOUNT_OBJECT from '@salesforce/schema/Account';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
// messageChannels
import {
subscribe,
unsubscribe,
MessageContext,
publish
} from "lightning/messageService";
import CART_CHANNEL from "@salesforce/messageChannel/productSelectedChannel__c";
import FILTER_CHANNEL from "@salesforce/messageChannel/productFilterChannel__c";
// apex
import getSelectedProducts from "@salesforce/apex/ProductService.getSelectedProducts";
import getAccount from "@salesforce/apex/ProductService.accountInfo";
import createRecord from "@salesforce/apex/ProductService.createOrderRecord";
import { createRecordInputFilteredByEditedFields } from "lightning/uiRecordApi";
export default class ProductCartList extends LightningElement {
subscription = null;
receivedMessage;
@track accountRecord = ACCOUNT_OBJECT;
@track addCartMap = {};
@track productWrap;
@track productList;
@track cartTitle ='Cart Details (0)';
@track isModalOpen = false;
@wire(MessageContext)
messageContext;
connectedCallback() {
this.subscribeMC();
}
disconnectedCallback() {
this.unsubscribeMC();
}
subscribeMC() {
if (this.subscription) {
return;
}
this.subscription = subscribe(
this.messageContext,
CART_CHANNEL,
(message) => {
console.log("message " + JSON.stringify(message));
this.addCartMap = message.selectedData;
this.findSelectedProducts();
}
);
}
unsubscribeMC() {
unsubscribe(this.subscription);
this.subscription = null;
}
findSelectedProducts() {
getSelectedProducts({productIdMap: this.addCartMap})
.then((result) => {
this.productWrap = result;
this.productList = result.productLineList;
this.cartTitle = 'Cart Details ('+result.count+')';
if(result.count <1){
this.productList = null;
}
})
.catch((error) => {
});
}
openModal() {
// to open modal set isModalOpen tarck value as true
this.isModalOpen = true;
}
closeModal() {
// to close modal set isModalOpen tarck value as false
this.isModalOpen = false;
this.accountRecord.Name = '';
this.accountRecord.Email__c = '';
this.accountRecord.BillingStreet = '';
this.accountRecord.BillingCity = '';
this.accountRecord.BillingState = '';
this.accountRecord.BillingCountry = '';
this.accountRecord.BillingPostalCode = '';
}
submitDetails() {
// to close modal set isModalOpen tarck value as false
//Add your code to call apex method or do some processing
if(this.checkIfError()){
var country = this.accountRecord.BillingCountry;
if(!country){
this.dispatchEvent(
new ShowToastEvent({
title: 'Error',
message: 'Please fill country field',
variant: 'error'
}),
);
} else {
createRecord({
acc: this.accountRecord,
productIdMap: this.addCartMap
})
.then(result => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Success',
message: 'Order created Successfully',
variant: 'success'
}),
);
this.closeModal();
this.publishChange();
this.addCartMap = {};
this.findSelectedProducts();
})
.catch(error => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Error creating record',
message: error.body.message,
variant: 'error'
}),
);
});
}
} else {
this.dispatchEvent(
new ShowToastEvent({
title: 'Error',
message: 'Please fill all required fields',
variant: 'error',
}),
);
}
}
findAccount() {
getAccount({emailId: this.accountRecord.Email__c})
.then((result) => {
if(result != null){
this.accountRecord.BillingStreet = result.BillingStreet;
this.accountRecord.BillingCity = result.BillingCity;
this.accountRecord.BillingPostalCode = result.BillingPostalCode;
this.accountRecord.BillingState = result.BillingState;
this.accountRecord.BillingCountry = result.BillingCountry;
}
})
.catch((error) => {
});
}
checkIfError(){
const isInputsCorrect = [...this.template.querySelectorAll('lightning-input')]
.reduce((validSoFar, inputField) => {
inputField.reportValidity();
return validSoFar && inputField.checkValidity();
}, true);
const isInputsCorrect1 = [...this.template.querySelectorAll('lightning-input-address')]
.reduce((validSoFar, inputField) => {
inputField.reportValidity();
return validSoFar && inputField.checkValidity();
}, true);
if(isInputsCorrect && isInputsCorrect1){
return true;
} else {
false;
}
}
addressInputChange( event ) {
this.accountRecord.BillingStreet = event.target.street;
this.accountRecord.BillingCity = event.target.city;
this.accountRecord.BillingState = event.target.province;
this.accountRecord.BillingCountry = event.target.country;
this.accountRecord.BillingPostalCode = event.target.postalCode;
console.log(event.target.street);
}
onChangeVal(event) {
if(event.target.name =='Name'){
this.accountRecord.Name = event.target.value;
} else if(event.target.name =='Email'){
this.accountRecord.Email__c = event.target.value;
this.findAccount();
}
}
publishChange() {
const message = {
filtersData: {
families: [],
minPrice: null,
maxPrice: null,
size: [],
brand: [],
color: [],
reset : true
}
};
publish(this.messageContext, FILTER_CHANNEL, message);
}
}
productCartList.html
<template>
<lightning-card title={cartTitle}>
<br/>
<template if:true={productList}>
<table class="slds-table slds-table_cell-buffer slds-table_bordered">
<thead>
<tr class="slds-line-height_reset">
<th class="" scope="col">
<div class="slds-truncate" title="Name">Product Name</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Quantity">Quantity</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Price">Price</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Total">Total</div>
</th>
</tr>
</thead>
<tbody>
<template for:each={productList} for:item="product">
<tr key={product.Id}>
<th scope="col">
<div>{product.productRecord.Name}</div>
</th>
<th scope="col">
<div> <lightning-formatted-number value={product.quantity}></lightning-formatted-number></div>
</th>
<th scope="col">
<div>
<lightning-formatted-number value={product.productRecord.Display_Price__c} format-style="currency"
currency-code="USD">
</lightning-formatted-number></div>
</th>
<th scope="col">
<div>
<lightning-formatted-number value={product.total} format-style="currency"
currency-code="USD">
</lightning-formatted-number></div>
</th>
</tr>
</template>
<tr></tr>
</tbody>
<tfoot>
<tr>
<td scope="col" colspan="3" style="text-align:right;"><b>Grand Total</b></td>
<td scope="col">
<div>
<lightning-formatted-number value={productWrap.total} format-style="currency"
currency-code="USD">
</lightning-formatted-number></div>
</td>
</tr>
</tfoot>
</table>
<br/>
<div style="text-align:center;">
<lightning-button variant="brand"
label="Make an Order"
title="Create Order"
onclick={openModal}
class="slds-m-left_x-small">
</lightning-button>
</div>
</template>
</lightning-card>
<template if:true={isModalOpen}>
<!-- Modal/Popup Box LWC starts here -->
<section role="dialog" tabindex="-1" aria-labelledby="modal-heading-01" aria-modal="true" aria-describedby="modal-content-id-1" class="slds-modal slds-fade-in-open">
<div class="slds-modal__container">
<!-- Modal/Popup Box LWC header here -->
<header class="slds-modal__header">
<button class="slds-button slds-button_icon slds-modal__close slds-button_icon-inverse" title="Close" onclick={closeModal}>
<lightning-icon icon-name="utility:close"
alternative-text="close"
variant="inverse"
size="small" ></lightning-icon>
<span class="slds-assistive-text">Close</span>
</button>
<h2 id="modal-heading-01" class="slds-text-heading_medium slds-hyphenate">Enter Order Details</h2>
</header>
<!-- Modal/Popup Box LWC body starts here -->
<div class="slds-modal__content slds-p-around_medium" id="modal-content-id-1">
<lightning-input autocomplete="false" message-when-value-missing="This is Required Field" class="inputfield" type="text" required="true" name="Name" label="Name" value={accountRecord.Name} onchange={onChangeVal} ></lightning-input>
<lightning-input autocomplete="false" message-when-value-missing="This is Required Field" class="inputfield" type="email" required="true" name="Email" label="E-mail" value={accountRecord.Email__c} onchange={onChangeVal} ></lightning-input>
<lightning-input-address class="inputfield" message-when-value-missing="This is Required Field"
address-label="Address"
street-label="Street"
street={accountRecord.BillingStreet}
city-label="City"
city={accountRecord.BillingCity}
country-label="Country"
country={accountRecord.BillingCountry}
province-label="State/ Province"
province={accountRecord.BillingState}
postal-code-label="Zip/ Postal Code"
postal-code={accountRecord.BillingPostalCode}
onchange={addressInputChange}
show-address-lookup required="true">
</lightning-input-address>
</div>
<!-- Modal/Popup Box LWC footer starts here -->
<footer class="slds-modal__footer">
<button class="slds-button slds-button_neutral" onclick={closeModal} title="Cancel">Cancel</button>
<button class="slds-button slds-button_brand" onclick={submitDetails} title="OK">Submit</button>
</footer>
</div>
</section>
<div class="slds-backdrop slds-backdrop_open"></div>
</template>
</template>
</template>
Please Provide MessageChannel File For This Code.