6. productTile: This component is responsible to show each product details. It’s getting product’s list from productList component and showing details about product.
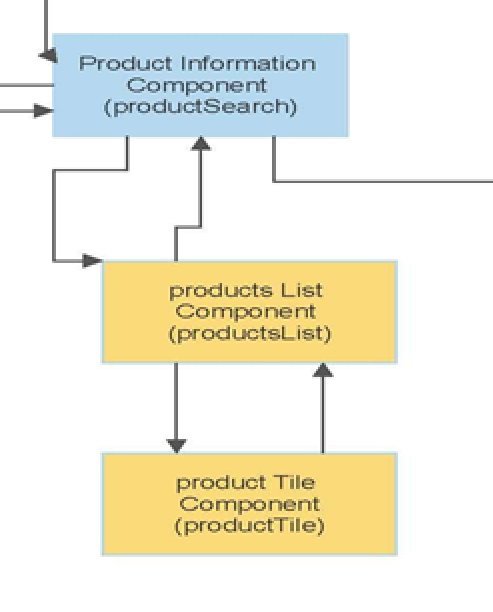
productTile.js
import { LightningElement, api } from "lwc";
export default class ProductTile extends LightningElement {
@api product;
isAddedToCart;
quantity;
@api
get addedToCart() {
return this.isAddedToCart;
}
set addedToCart(value) {
this.isAddedToCart = value;
}
@api
get defaultQuantity() {
return this.quantity;
}
set defaultQuantity(value) {
this.quantity = value;
}
handleAddToCart() {
this.isAddedToCart = true;
this.dispatchEvent(
new CustomEvent("addedtocart", {
detail: {
productId: this.product.Id,
selectedQuantity: this.quantity
}
})
);
}
handleRemoveFromCart() {
this.isAddedToCart = false;
this.dispatchEvent(
new CustomEvent("removedfromcart", {
detail: {
productId: this.product.Id
}
})
);
this.quantity = 1;
}
handleChange(event) {
this.quantity = event.target.value;
if(this.isAddedToCart == true){
this.handleAddToCart();
}
}
get backgroundStyle() {
return `background-image:url(${this.product.ImageUrl__c})`;
}
get totalPrice() {
return this.quantity * this.product.Display_Price__c;
}
}
productTile.html
<template>
<div class="slds-var-p-around_small">
<div style={backgroundStyle} class="slds-container_fluid image"></div>
<h1 class="slds-var-p-around_xx-small slds-var-m-top_x-small slds-truncate slds-text-title_caps bold-text">
{product.Name}
</h1>
<h2 class="slds-var-p-around_xx-small slds-text-body_regular">
<lightning-layout>
<lightning-layout-item size="6" class="slds-var-p-right_xx-small">
Price:
<lightning-formatted-number value={product.Display_Price__c} format-style="currency"
currency-code="USD">
</lightning-formatted-number>
</lightning-layout-item>
<lightning-layout-item size="6" class="slds-var-p-right_xx-small bold-text">
Total:
<lightning-formatted-number value={totalPrice} format-style="currency" currency-code="USD">
</lightning-formatted-number>
</lightning-layout-item>
</lightning-layout>
</h2>
<!-- <div class="slds-var-p-around_xx-small slds-text-body_small">
<lightning-formatted-rich-text value={product.description}>
</lightning-formatted-rich-text>
</div> -->
<div class="slds-var-p-around_xx-small slds-text-body_small slds-container_fluid">
<lightning-layout>
<lightning-layout-item size="6" class="slds-var-p-right_xx-small">
<lightning-input step="1" variant="label-hidden" type="number" value={quantity} label="Quantity"
onchange={handleChange}>
</lightning-input>
</lightning-layout-item>
<lightning-layout-item size="6" class="slds-var-p-left_xx-small">
<lightning-button if:false={isAddedToCart} variant="Brand" label="Add to cart" title="Add to cart"
onclick={handleAddToCart}>
</lightning-button>
<lightning-button if:true={isAddedToCart} variant="destructive" label="Remove" title="Remove"
onclick={handleRemoveFromCart}>
</lightning-button>
</lightning-layout-item>
</lightning-layout>
</div>
</div>
</template>
productTile.css
.image {
height: 250px;
background-position: center;
background-size: cover;
border-radius: 5px;
padding: var(--lwc-borderWidthThin, 1px) !important;
}
.bold-text {
font-weight: bold;
}